Monospaced 11x10 Font
For my VT100 terminal emulator on Raspberry Pi Pico project I needed to make my own font to fit the specific requirements. For convenience, that source code only contains the font data as a static "char.rom" binary file. For completeness sake I present the font data source here.
The source data is an image, so any modifications can be done in an image editor:
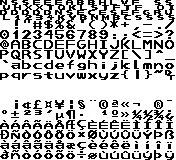
As can be seen here the image is divided into a grid of 16x16 to provide 256 characters in total.
This image needs to be converted to PGM format and then be fed into a special utility that converts it to the actual ROM binary file.
The source code for the PGM to ROM utility is provided here, which operates on stdin/stdout for the conversion:
#include <stdio.h> #include <stdbool.h> #define HEIGHT 10 #define WIDTH 11 #define CHAR_PER_LINE 16 #define BYTES_PER_LINE 176 #define COLLECT (HEIGHT * BYTES_PER_LINE) static unsigned char buffer[COLLECT]; static int power_of_two(int n) { if (n == 0) { return 1; } else { return 2 * power_of_two(n - 1); } } int main(void) { int skip = 4; int c, n, i, j, k; int b; n = 0; while ((c = fgetc(stdin)) != EOF) { if (skip > 0) { if (c == '\n') { skip--; } continue; } buffer[n] = c; n++; if (n >= COLLECT) { n = 0; /* Store as 16 bits by 10 lines, even if 11 bits wide. */ for (i = 0; i < CHAR_PER_LINE; i++) { for (j = 0; j < HEIGHT; j++) { b = 0; for (k = 0; k < 8; k++) { if (buffer[k + (i * WIDTH) + (j * BYTES_PER_LINE)] == 0) { b += power_of_two(7 - k); } } fputc(b, stdout); b = 0; for (k = 0; k < 3; k++) { if (buffer[k + 8 + (i * WIDTH) + (j * BYTES_PER_LINE)] == 0) { b += power_of_two(2 - k); } } fputc(b, stdout); } } } } return 0; }