Byte Value Visualization
While doing cryptanalysis on some XOR-encrypted files, I came up with the idea of visualizing byte values in these files in order to discover patterns. Having used the SDL library on previous projects, this was a good candidate to draw the graphics.
Here is an output example, visualizing the source code of this application:
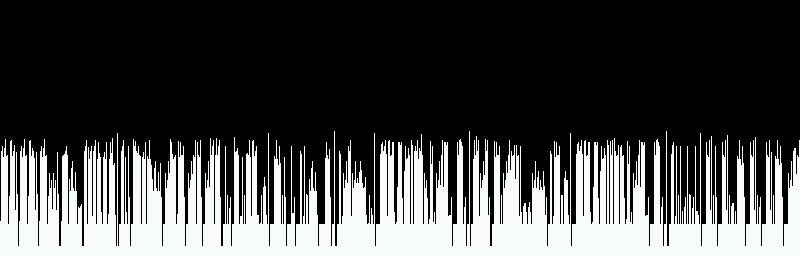
It is easy to see that this is a plain text file, since there are mostly printable ASCII characters in the 0x20 to 0x7F area. You can also see the low-value newline characters (0x0A) clearly.
Here is another example, visualizing the application in binary form after compilation:
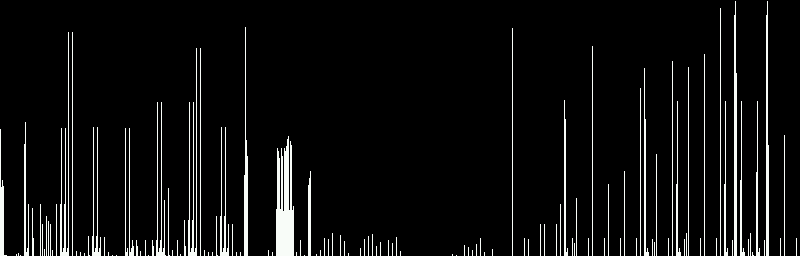
This is clearly not human-readable text, as there is a lot of NULL (0x00) bytes, and some bytes with very high values (0xFF).
I believe it's a lot easier to spot patterns this way, instead of using a hex editor. The application reads data (byte values) from standard in and stops after 1024 bytes or EOF.
But enough talk, here is the source code:
#include <stdio.h> #include <stdlib.h> #include <SDL/SDL.h> #define MAX_DATA 1024 int main(int argc, char *argv[]) { int c, n; unsigned char data[MAX_DATA]; SDL_Surface *screen; SDL_Rect rect; SDL_Event event; n = 0; while ((c = fgetc(stdin)) != EOF) { data[n] = c; n++; if (n >= MAX_DATA) break; } if (SDL_Init(SDL_INIT_VIDEO) != 0) { fprintf(stderr, "Error: Unable to initalize SDL: %s\n", SDL_GetError()); return 1; } atexit(SDL_Quit); screen = SDL_SetVideoMode(n, 255, 16, SDL_DOUBLEBUF); if (screen == NULL) { fprintf(stderr, "Error: Unable to set video mode: %s\n", SDL_GetError()); return 1; } for (n = n - 1; n >= 0; n--) { rect.x = n; rect.y = 255 - data[n]; rect.w = 1; rect.h = data[n]; SDL_FillRect(screen, &rect, 0xffffff); } SDL_Flip(screen); while (1) { if (SDL_PollEvent(&event) == 1) { if (event.type == SDL_KEYDOWN) { if (event.key.keysym.sym == SDLK_q) break; } else if (event.type == SDL_QUIT) { break; } } SDL_Delay(50); } return 0; }