Sharp IQ-7100M Linux Link
Here is a way to download data from a Sharp IQ-7100M organizer into a modern Linux system. To be able to make the connection you will need to use a USB<->UART converter that operates on a lower TTL voltage level and most importantly INVERTS the TXD and RXD logic levels. I used the SparkFun DEV-09873 which is actually 3.3V, but the 5V version probably works as well since the Sharp organizer uses 5V TTL levels. The FTDI FT232RL chip on this supports inverting the TXD and RXD logic levels by using the "FT_PROG" program to reprogram the settings.
The connection table is as follows:
|------|--------------| | FTDI | Sharp 15-Pin | | -----|---|----------| | GND | 7 | GND | | RXD | 2 | TXD | | TXD | 3 | RXD | |------|---|----------|
With the SparkFun adapter it is possible to just use three wires with male Dupont connectors:
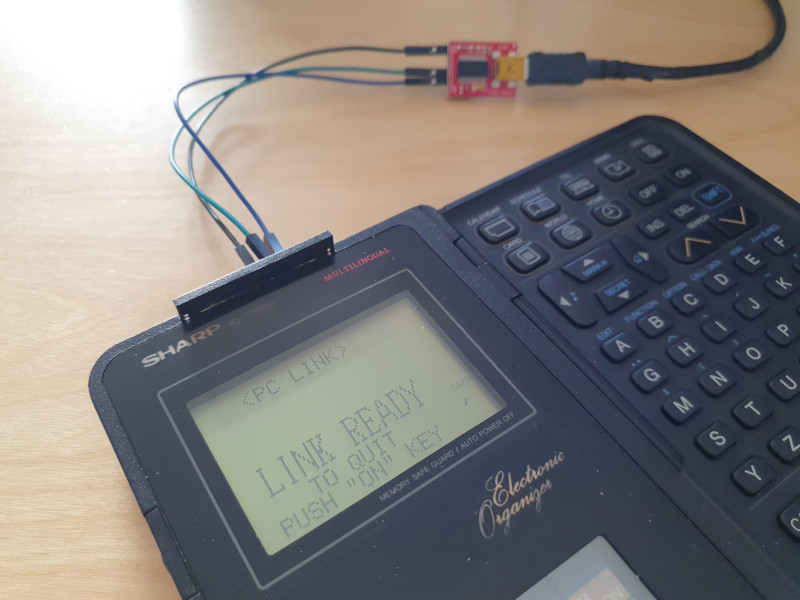
Here is a small C program that will download the "MEMO" database:
#include <stdlib.h> #include <stdio.h> #include <errno.h> #include <unistd.h> #include <fcntl.h> #include <termios.h> #define TTY_DEVICE "/dev/ttyUSB0" static const char command[] = "\x04R030000\r\nMEMO 1 \r\n"; int main(void) { int fd; struct termios tios; unsigned char byte; const char *p; fd = open(TTY_DEVICE, O_RDWR | O_NOCTTY); if (fd == -1) { fprintf(stderr, "open() failed with errno: %d\n", errno); return EXIT_FAILURE; } if (tcgetattr(fd, &tios) == -1) { fprintf(stderr, "tcgetattr() failed with errno: %d\n", errno); close(fd); return EXIT_FAILURE; } cfmakeraw(&tios); cfsetispeed(&tios, B9600); cfsetospeed(&tios, B9600); if (tcsetattr(fd, TCSANOW, &tios) == -1) { fprintf(stderr, "tcsetattr() failed with errno: %d\n", errno); close(fd); return EXIT_FAILURE; } /* Send the command, using a small delay: */ p = &command[0]; while (*p != '\0') { if (write(fd, p, 1) == -1) { fprintf(stderr, "write() failed with errno: %d\n", errno); close(fd); return EXIT_FAILURE; } usleep(10000); p++; } /* Read the response until a EOF character appears: */ do { if (read(fd, &byte, 1) != 1) { fprintf(stderr, "read() failed with errno: %d\n", errno); close(fd); return EXIT_FAILURE; } putc(byte, stdout); } while (byte != 0x1A); close(fd); return EXIT_SUCCESS; }
This program just dumps the raw data, which is already in ASCII. Since there is no flow control, a sleep of 10ms was added between each byte sent to prevent the Sharp organizer from overflowing and giving up. Note that you will need to be in the "PC-Link" menu for any transfer to work, which is reached by pressing "Shift" + "Option" then "4".