Steam Status on LCD
I recently provided code to drive a certain LCD under Linux. Currently, I use it to monitor my friends activites on the Steam network, that are used for games like Team Fortress 2. The steam status information is gathered with Perl script that downloads a public steam webpage every third minute. Afterwards the information is parsed and the formatted output is passed to the LCD display through a userland program.
The final output looks like this:
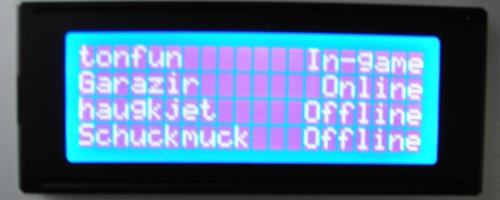
The Perl code:
#!/usr/bin/perl -w use strict; use LWP::Simple; my $steam_id = "your_steam_id_here"; my $url = "http://steamcommunity.com/id/$steam_id"; while (1) { my $content = get($url); if (defined $content) { my (@friend, @status); while ($content =~ m/<p><a class="linkFriend_([^"]+)"[^>]+?>([^<]+)</g) { push @status, ucfirst($1); push @friend, $2; } for (my $i = 0; $i < 4; $i++) { if (defined $friend[$i] and defined $status[$i]) { # Status should not be longer than 7 characters. Could fit a name # of 13 characters, but keep an extra space for clarity. system("./lcd-putrow " . ($i + 1) . " \'" . (sprintf "%-13s", substr($friend[$i], 0, 12)) . (sprintf "%7s", $status[$i]) . "\'"); } } } else { system("./lcd-putrow 1 \' \'"); system("./lcd-putrow 2 \' SERVER\'"); system("./lcd-putrow 3 \' UNAVAILABLE\'"); system("./lcd-putrow 4 \' \'"); } sleep(180); }